- Introduction
- What is React?
- Why Use React?
- Benefits of React for Front-End Development
- Real-World Examples of React in Action
- Conclusion
- Frequently Asked Questions (FAQs)
Table of Contents
The Benefits of Using React for Front-End Development
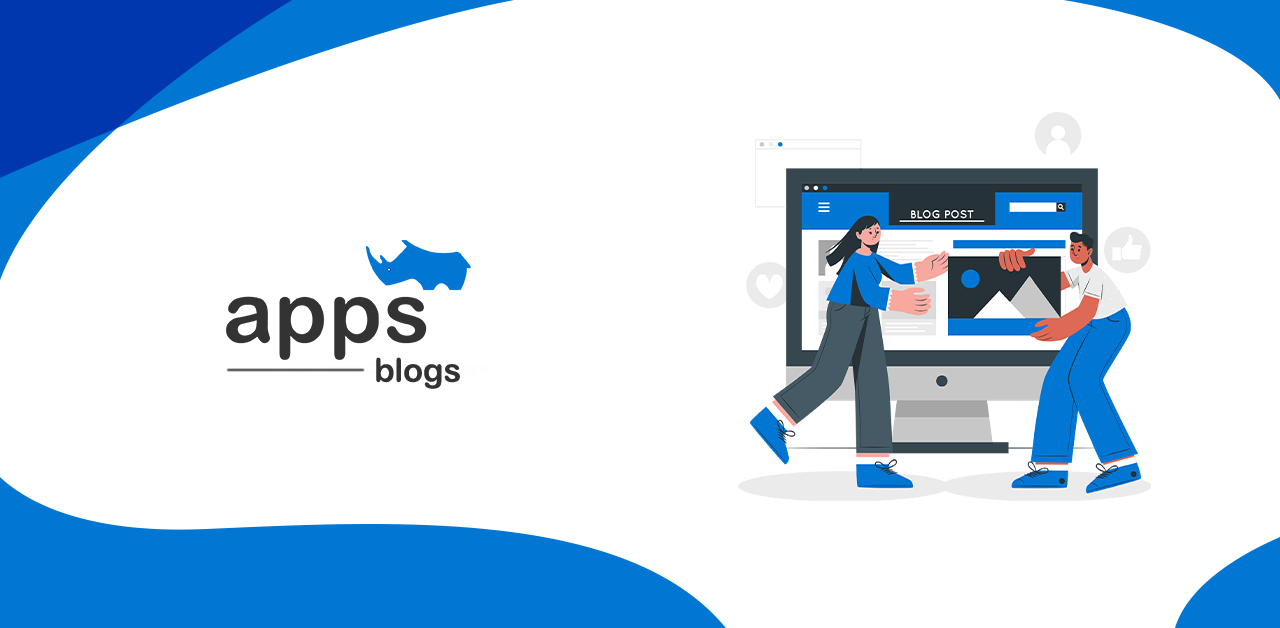
Introduction
Curious about why developers are buzzing about React for front-end development? You've landed in the right place. Here, we keep the tech jargon at bay and focus on simple language. So, let's begin by understanding what React actually is.
React is a JavaScript library loved globally for its proficiency in building user interfaces. It’s not just a trendy tool, but one that handles complexities of coding in an efficient way.
But that's not all; React does wonders for app performance too. It directly translates to a refined and smooth user experience, something every good app strives for.
But the list of benefits doesn't end here. There's much more to React, like its component-based structure and strong community support.
Stick along as we delve into why React has gained such popularity among developers. Without any fluff or complex terms, we promise you a straightforward rundown of React's undeniable benefits. Let's dive in!
What is React?
React is a JavaScript library for which is used for the structuring of user interfaces. It was laid down by Facebook in 2011 and since then has revolutionized itself as the most well known front-end development device to exist. React allows developers to put reusable UI components and handle the state of their application with ease.
React's Architecture
React's architecture follows the concept of a virtual DOM (Document Object Model). The virtual DOM is a lightweight representation of the actual DOM, permitting React to upgrade the user interface skillfully. When a change has occurred in the state of a component, React updates the virtual DOM and then compares it to the actual DOM. Only the fragments of the actual DOM which are required to be updated are changed, resulting in faster and more efficient updates.
React's Virtual DOM
An important characteristic of React is its virtual DOM. This particular feature of React distinguishes it from other front-end development tools. The virtual DOM authorizes lightning-fast updates to the user interface resulting in a smoother user experience. Since React only updates the parts of the DOM that have changed, rather than re-rendering the entire page.
Why Use React?
Now that we know what React is, let's explore why it's such a popular choice among developers.
Improved Performance
As discussed above, React's virtual DOM enhances quicker and well organized updates to the user interface. Hereby resulting in better performance and a smoother user experience.
Reusable Components
The component-based architecture of React permits developers to build reusable UI components. Meaning that developers can formulate a component once and use it throughout their application, rather than having to write the same code multiple times. Henceforth saving time and also making it easier to handle and update the application.
Easy to Learn and Use
One of the most important features due to which React is known is its easy to learn and use aspect even for developers who are new to front-end development. React uses a simple syntax called JSX, which permits developers to write HTML-like code within their JavaScript files.
Large Community Support
A vast and ever active community of developers is supplied from React’s end to its users who are readily available to help out. Meaning that if you come across any issues or questions, you can easily find answers and support online.
SEO-Friendly
Developers often stress on checking if their website is SEO-friendly or not. With React , it is done at ease as it allows for easy optimization of your site for search engines. React's server-side rendering capabilities permits search engines to crawl and index your site more easily, subjecting it to better search engine rankings.
How to Use React
Using React can seem daunting at first, but it's actually quite straightforward. Here are the basic steps for setting up a React project:
Setting up a React Project
- Install Node.js and npm: Node.js is a JavaaScript runtime that allows you to run JavaScript on the server-side. npm is a package manager for Node.js that allows you to install and manage packages.
- Create a new project directory: This is where you'll store all of your project files.
- Initialize a new npm project: This creates a package.json file, which contains information about your project and its dependencies.
- Install React and React DOM: React is the core library, while React DOM is used for rendering React components in the browser.
- Create a new index.html file: This is the main HTML file for your project.
- Create a new index.js file: This is the main JavaScript file for your project.
- Add the React code to your index.js file: This is where you'll write your React code.
- Run your project using npm start: This will start a development server and open your project in the browser.
Creating Components
Creating components is one of the most important parts of building a React application. Here's how to create a basic component:
- Create a new file for your component: This is where you'll store your component code.
- Import React and the component class: This allows you to use React and the component class in your component.
- Define your component class: This is where you'll define the structure and behavior of your component.
- Add your component's render method: This is where you'll define what your component should render.
- Export your component: This allows you to use your component in other parts of your application.
Using JSX
JSX is a syntax extension for JavaScript permitting you to write HTML-like code in your JavaScript files.
Here's an example of how to use JSX:
jsx
const element = <h1>Hello, world!</h1>;
Managing State and Props
The two chief concepts in React are State and props. State is utilized in managing the interior state of a component, while props are used to pass data from one component to another.
Here's an example of how to use state and props:
jsx
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
handleClick() {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={() => this.handleClick()}>Click me</button>
</div>
);
}
}
Handling Events
Handling events in React and vanilla JavaScrip is almost the same. Here's an example of how to handle a click event:
jsx
class MyComponent extends React.Component {
handleClick() {
console.log('Button clicked');
}
render() {
return (
<button onClick={() => this.handleClick()}>Click me</button>
);
}
}
Benefits of React for Front-End Development
React is a powerful and versatile tool for front-end development. Here are just a few of the benefits that React offers:
Improved Code Organization
React's component-based architecture helps in organizing your code. This is done by segregation of your application into tinier, reusable components. So that the code can be kept organized and therefore will be much easier to handle. Larger projects benefit from them because keeping track of all your code becomes a challenge.
Faster Development Time
The reusable components and easy-to-learn syntax of React yield in faster development of applications. Meaning that you can structure high-quality user interfaces in less time, which can be a huge advantage in today's fast-paced development environment. This aids in getting your product to market faster, which can be a key factor in the success of your project.
Better User Experience
React's virtual DOM and fast updates results in a smoother user experience. You get to collaborate with your application readily and efficiently, resulting in a better overall experience. This is extremely crucial for applications where speed and responsiveness are key, such as e-commerce sites or social media platforms.
Cross-Platform Compatibility
React can be utilized in the formation of applications for a variety of platforms, including web, mobile, and desktop. Meaning that React can be put to use for the formation of applications that work across multiple platforms saving time and resources in the process. This can be especially helpful for businesses that want to reach a wide audience with their products or services.
Easy Integration with Other Libraries and Frameworks
React can be easily integrated with other libraries and frameworks, such as Redux and React Native. This means that you can use React in conjunction with other tools to build even more powerful applications. This can be especially helpful for developers who want to take advantage of the strengths of different tools to build the best possible application.
Real-World Examples of React in Action
A number of well known websites and applications including Facebook, Instagram, Airbnb, and Netflix use React in one way or the other .
Facebook applies React to power its news feed, which is known to be the most complex user interface on the web. The component-based architecture of React assists Facebook in handling various elements of the news feed, such as posts, comments, and reactions.
React is utilized by Instagram in order to power its web application, allowing users to browse and interconnect with their Instagram feeds. The fast updates and virtual DOM provided by React eases the responsive user experience, which is extremely important for a social media platform like Instagram.
Airbnb
For powering its search and booking interfaces Airbnb incorporates the features of React , since it is crucial for the success of the company. The reusable components and easy-to-learn syntax of React make it easy for Airbnb to build and maintain these interfaces, which are key to the user experience of the platform.
Netflix
In order to power its user interface Netflix takes the help of React, this permits users to look around and watch their favorite movies and TV shows. React's fast updates and virtual DOM make for a seamless and responsive user experience, which is essential for a streaming platform like Netflix.
Conclusion
Lastly, React is a trustworthy and flexible device well fitted for front-end development. The component-basaed architecture, fast updates, and easy-to-learn syntax acts as a contributing factor on keeping React as the most desired among developers. Many well known websites and applications use React including Facebook, Instagram, Airbnb, and Netflix, which is a testament to its effectiveness and versatility.
Whether you're building a simple website or a complex web application, React is definitely worth considering. So why not give it a try? With its many benefits and real-world examples of)
success, React is sure to be a valuable tool in your front-end development toolkit.
Frequently Asked Questions (FAQs)
What is React and how is it used for front-end development?
React is an open-source JavaScript library used for building user interfaces. It is used for front-end development to create dynamic and responsive web applications that can handle large amounts of data and complex user interactions.
What are the benefits of using React for front-end development?
React offers many benefits for front-end development, including faster load times, smoother performance, and reusable code. It also allows developers to create a modular and efficient code structure, making it easier to maintain and update applications.
How is React different from other front-end frameworks like Angular or Vue.js?
React differs from other front-end frameworks in several ways, such as its focus on component-based development, its use of a virtual DOM, and its emphasis on declarative programming. Additionally, React is generally considered more lightweight and flexible than other frameworks.
Is React suitable for large-scale front-end development?
Yes, React is suitable for large-scale front-end development, as it provides a scalable and efficient way to build complex web applications. It has been used by many large-scale companies and websites, such as Facebook, Airbnb, and Netflix.
What are some of the challenges of using React for front-end development?
While React offers many benefits, there are also some challenges to using it for front-end development. One potential challenge is the learning curve, as React has a unique syntax and structure that might be unfamiliar to some developers. Additionally, React's frequent updates and changes may require ongoing training and support.
Table of Contents
- Introduction
- What is React?
- Why Use React?
- Benefits of React for Front-End Development
- Real-World Examples of React in Action
- Conclusion
- Frequently Asked Questions (FAQs)