- Introduction
- What is Java Spring Boot?
- Benefits of using Java Spring Boot for web application development
- Setting up a Java Spring Boot project
- Building RESTful APIs with Java Spring Boot
- Working with Databases in Java Spring Boot
- Securing Java Spring Boot Applications
- Securing RESTful APIs with JSON Web Tokens (JWT)
- Integrating front-end frameworks with Java Spring Boot
- Conclusion
- Frequently Asked Questions (FAQs)
Table of Contents
Java Spring Boot Developers: Build Powerful Web Applications
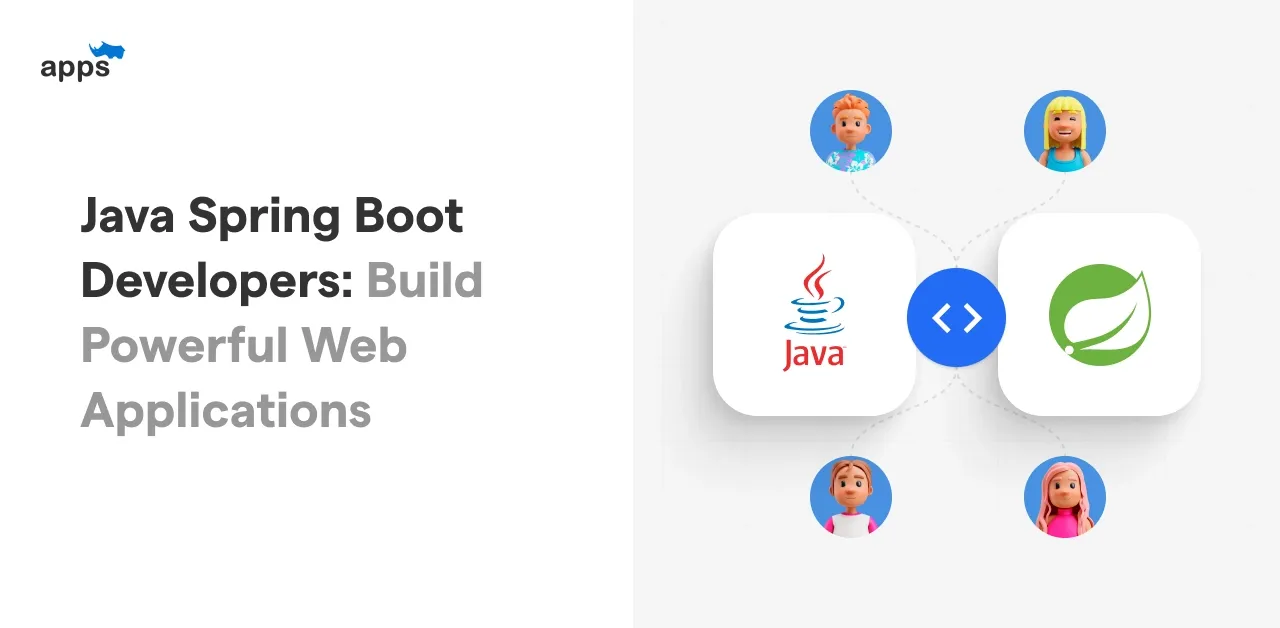
Introduction
The world of web applications have been altered by Java Spring Boot Developers with their robust and streamlined framework. Keeping the current digital age in mind, the presence of web applications have become the backbone of businesses, providing everything from e-commerce platforms to social media networks.
Java Spring Boot developers are at the soldiers of this technological evolution, as they possess the skills to build powerful and efficient web applications.
This guide explores the significance of Java Spring Boot developers in today’s software landscape, emphasizing their role in creating powerful and efficient web applications that drive innovation and productivity. By harnessing the capabilities of Spring Boot, these developers are equipped to meet the demands of modern web development, delivering dynamic and scalable solutions for a wide array of industries.
What is Java Spring Boot?
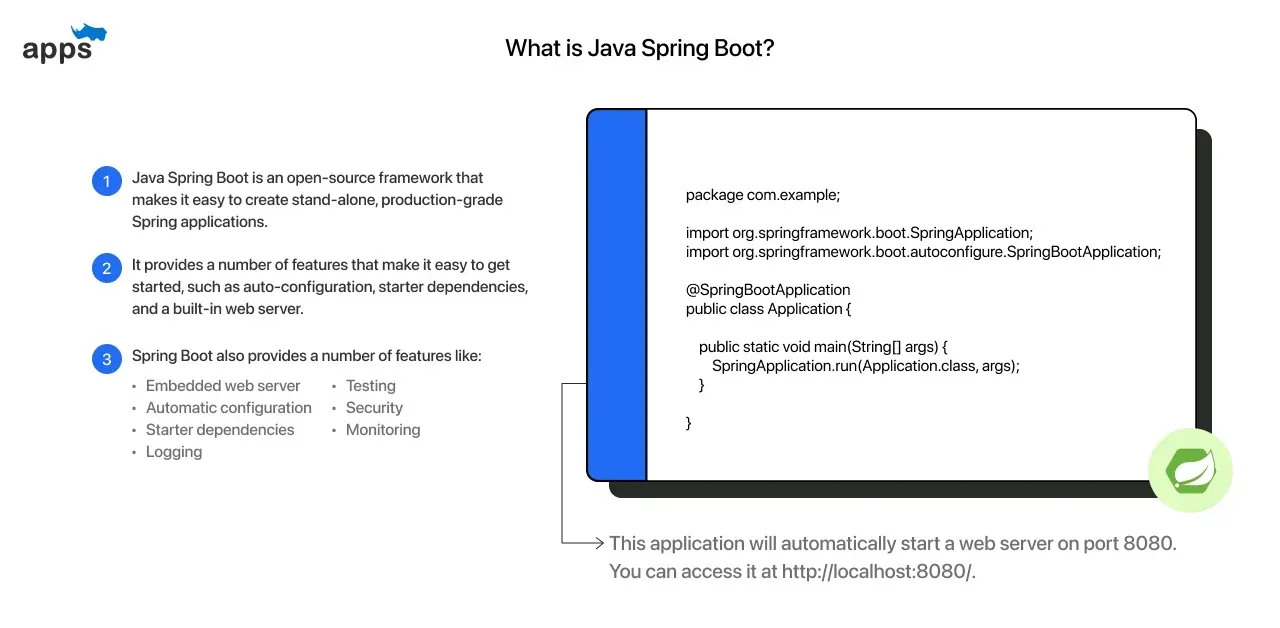
Java Spring Boot is a framework designed to simplify the development of Java-based web applications.
It takes the Spring framework's core features and combines them with opinionated conventions and auto-configuration to provide a streamlined development experience.
Spring Boot eliminates much of the configuration overhead usually associated with Spring applications, allowing developers to focus more on business logic and application features.
Benefits of using Java Spring Boot for web application development
- Increased productivity: Spring Boot's opinionated approach reduces the amount of boilerplate code, making development faster and more efficient.
- Easy setup and deployment: Spring Boot provides a command-line interface and a starter project structure that facilitates the setup and deployment of Java Spring Boot applications.
- Auto-configuration: Spring Boot automatically configures the application based on sensible defaults, significantly reducing the need for manual configuration.
- Integrated dependencies: Spring Boot manages project dependencies, resolving conflicts and ensuring compatibility between libraries used in the application.
- Simplified testing: Spring Boot offers convenient testing support, including the ability to launch the application in an embedded container for integration testing.
- Production-ready features: Spring Boot provides built-in features for monitoring, metrics, and health checks, making it easier to manage and monitor applications in production.
- Large ecosystem and community support: Spring Boot leverages the broader Spring ecosystem and has a large and active community, offering a wealth of resources, plugins, and community-driven projects.
Setting up a Java Spring Boot project
Let’s see how you can setup a Java Spring Boot on your system:
Installing Java and Spring Boot
Explaining the steps to install the Java Development Kit (JDK) on various platforms (e.g., Windows, macOS, Linux).
Providing instructions to set up the Java development environment and verify the installation.
Guiding readers on how to install Spring Boot using tools like Spring Initializr or through build automation tools like Maven or Gradle.
Creating a new Java Spring Boot project
There are different options for creating a new Spring Boot project. These options range from using Spring Initializr, IDE integrations (e.g., IntelliJ IDEA, Eclipse), or command-line tools.
In order to create a Java Spring boot project developers first need to know how to create a new project, choose the desired project structure, and configure initial project settings.
Configuring project dependencies and libraries
- Project dependencies can be managed by using build automation tools like Maven or Gradle.
- Spring Boot incorporates a concept of starter dependencies which can simplify the management of common dependencies required for specific functionalities (e.g., web applications, database access).
- Developers can add and configure additional dependencies (e.g., for specific databases, security frameworks) based on project requirements and desired functionalities.
- By following these steps, developers can set up a Java Spring Boot project, ensuring they have the necessary tools and dependencies to start building powerful web applications efficiently.
Building RESTful APIs with Java Spring Boot
Next on the line is Building RESTful APIs. Let’s see how it can be done with Java Spring Boot:
Introduction to RESTful architecture
Briefly explaining the principles and concepts of the Representational State Transfer (REST) architecture.
Discussing RESTful design principles, including resource identification, statelessness, and the use of HTTP methods (GET, POST, PUT, DELETE) for CRUD operations.
Highlighting the benefits of using RESTful APIs for building web services.
Creating RESTful endpoints and handling HTTP requests
The ways to create endpoints in Spring Boot and handle HTTP requests are:
- Annotations can be put to use to define endpoints and map them to specific URLs. This can be done in ways such as @RestController, @RequestMapping, and @GetMapping to define endpoints and map them to specific URLs.
- Different types of HTTP requests can be handled in various ways for example (GET, POST, PUT, DELETE) and their corresponding API operations.
Suggested Reading:
The Best Tools for Successful Java Spring Boot Development
Implementing CRUD operations with Java Spring Boot
The implementation of CRUD (Create, Read, Update, Delete) operations using Spring Boot can be done by.
- The appropriate use of HTTP methods and request mapping annotations to handle each operation.
- Developers can process Request parameters, request bodies, and path variables to perform the desired CRUD actions.
Working with Databases in Java Spring Boot
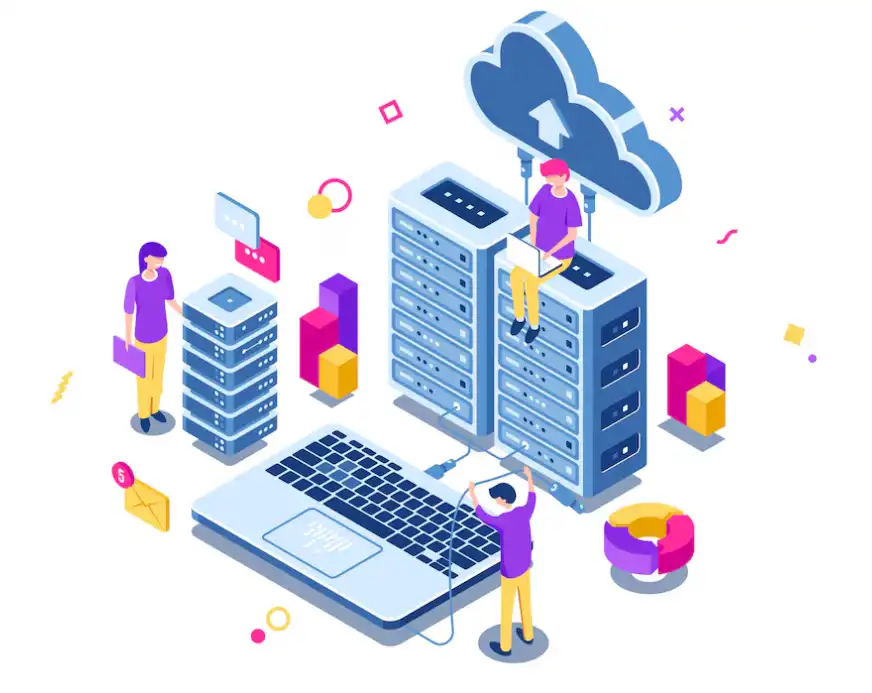
How to work with databases in Java Spring Boot, you ask? Here’s what you need to do:
Configuring database connections
Discussing the various options for configuring database connections in Spring Boot, including configuring a connection to a relational database (e.g., MySQL, PostgreSQL) through application.properties or application.yml, or using environmental variables.
Providing step-by-step instructions on how to set up and configure the database connection.
Performing CRUD operations with Java Spring Boot and JPA
The concept of the Java Persistence API (JPA) is used in Spring Boot to simplify database operations. It is also used to define entities and repositories using JPA annotations. At times repository methods can also be put to use to perform CRUD operations, such as saving entities, retrieving entities by ID, updating entities, and deleting entities.
Implementing database migrations
Database migrations are extremely important in terms of building powerful web applications. Their major role revolves around maintaining the consistency of database schema over time. Tools like Flyway or Liquibase that can be used to manage database migrations in Spring Boot. These tools can be used to modify, evolve the database schema. The creation and application of database migration scripts can also be implemented
By understanding and implementing these concepts and techniques, developers can effectively build RESTful APIs and interact with databases in Java Spring Boot applications.
Securing Java Spring Boot Applications
Unlock the essential practices and strategies to safeguard your applications in today’s dynamic cybersecurity environment.
Authentication and authorization with Spring Security
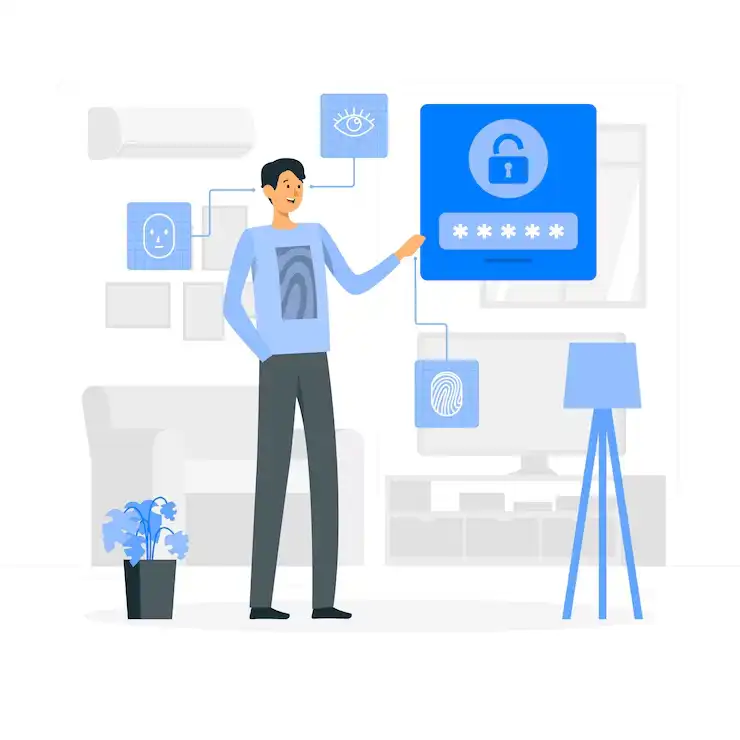
Authentication refers to the process of verifying the identity of users attempting to access an application. Authorization, on the other hand, involves determining what actions an authenticated user is allowed to perform within the application. Spring Security is a powerful framework that provides a comprehensive set of features for securing Java Spring Boot applications.
To implement authentication with Spring Security, developers can configure various authentication mechanisms such as form-based login, OAuth, or LDAP. This allows users to provide their credentials and gain access to the application. Spring Security handles the authentication process and provides a way to customize it to fit the specific requirements of the application.
Authorization in Spring Security involves defining roles and permissions for users. Role-based access control (RBAC) is commonly used, where users are assigned roles that determine their level of access within the application. Developers can configure Spring Security to enforce access control rules based on the roles of users. This ensures that only authorized users can perform certain actions or access specific resources.
Implementing role-based access control
Role-based access control (RBAC) is a widely adopted approach to implementing access control in applications. With RBAC, users are assigned roles that determine the actions they can perform and the resources they can access within the application. Spring Security provides features to implement RBAC in Java Spring Boot applications.
To implement RBAC with Spring Security, developers can configure roles and permissions for users. Roles can be defined for different user types or job positions, such as "admin", "user", or "manager". Permissions define the specific actions that users with certain roles can perform. For example, an admin role might have the permission to create, update, and delete resources, while a user role might only have permission to read resources.
Spring Security provides annotations and expressions that can be used to enforce access control rules based on user roles and permissions. Developers can annotate methods or endpoints with @PreAuthorize or @Secured annotations to specify the roles or permissions required to access them. This ensures that only authorized users can perform certain actions or access specific resources within the application.
Suggested Reading:
Top 7 Qualities of an Outstanding Java Spring Boot Developer
Securing RESTful APIs with JSON Web Tokens (JWT)
JSON Web Tokens (JWT) have become a popular way to secure RESTful APIs. JWT is a compact and self-contained token format that can be used to authenticate and authorize API requests. Spring Security provides support for integrating JWT authentication and authorization mechanisms into Java Spring Boot applications.
To secure RESTful APIs with JWT, developers can configure Spring Security to generate and validate JWTs. When a user logs in or authenticates, a JWT is generated and returned to the client. This JWT contains necessary information such as the user's identity and roles. The client then includes this JWT in subsequent API requests, typically in the Authorization header.
Spring Security provides mechanisms to validate and parse the JWT on the server-side. It verifies the signature of the JWT and extracts the necessary information to authenticate and authorize the API request. The user's roles and permissions can be retrieved from the JWT and used to enforce access control rules.
Integrating front-end frameworks with Java Spring Boot
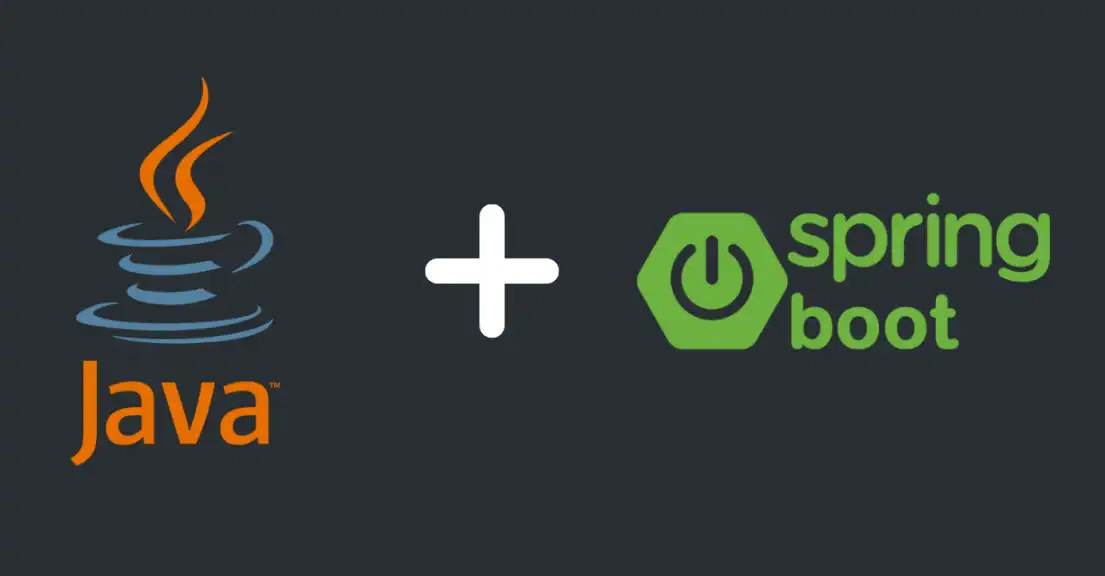
Discover how this integration elevates user-experiences while maintaining the efficiency and scalability synonymous with Java Spring Boot’s backend powers.
Using Thymeleaf for server-side rendering
Thymeleaf is a server-side templating engine that integrates well with Java Spring Boot for rendering dynamic web pages on the server. With Thymeleaf, developers can create templates that include both static content and dynamic data that is populated by the server.
To use Thymeleaf, developers define HTML templates with Thymeleaf-specific attributes and syntax. These templates can include placeholders for dynamic data, which is populated by the server before rendering the page. Thymeleaf provides a set of predefined expressions and tags that can be used to access and display data from the backend, perform conditional rendering, iterate over collections, and more.
In a Java Spring Boot application, developers can configure Thymeleaf as the template engine and define controller methods that return the name of the Thymeleaf template to be rendered. The controller can also pass data to the template, which can be accessed and displayed using Thymeleaf expressions.
Building a RESTful API-driven front-end with Angular or React
Angular and React are popular front-end frameworks that allow developers to build powerful single-page applications (SPAs). These frameworks can be used to create a user interface that consumes RESTful APIs provided by a Java Spring Boot backend.
With Angular or React, developers can create components that interact with the backend API. These components can include forms, tables, and other UI elements that allow users to interact with the API and display data retrieved from the server.
To integrate Angular or React with a Java Spring Boot backend, developers set up a project for the front-end framework and configure it to make HTTP requests to the backend API. The front-end code can include service classes or API wrappers that encapsulate API calls and handle responses. These classes can be used to retrieve data from the backend, send data for processing, or perform other actions on the server.
Consuming APIs from a Java Spring Boot backend
Consuming APIs from a Java Spring Boot backend involves making HTTP requests to the API endpoints provided by the backend. This allows front-end applications or other external systems to retrieve data, send data for processing, or interact with the backend in various ways.
To consume APIs from a Java Spring Boot backend, developers can use libraries or frameworks that simplify the process of making HTTP requests. For example, libraries like Retrofit or Feign provide a high-level API that allows developers to define API interfaces and make API calls using simple method invocations.
By defining API interfaces, developers can specify the URL, request parameters, headers, and other details for each API endpoint. The library handles the low-level details of making the HTTP request, serializing/deserializing JSON, and processing the response. This allows developers to focus on using the data returned by the API to update the UI or perform other actions.
Overall, securing Java Spring Boot applications involves implementing authentication and authorization mechanisms, such as Spring Security, to control access to resources. Additionally, integrating front-end frameworks like Thymeleaf, Angular, or React allows for the creation of user-friendly interfaces that consume APIs from the Java Spring Boot backend.
Conclusion
In this blog, we explored various aspects of securing, integrating, and deploying Java Spring Boot applications. We discussed authentication and authorization with Spring Security, implementing role-based access control, securing RESTful APIs with JWT, and integrating front-end frameworks like Thymeleaf, Angular, or React.
Additionally, we touched on packaging and deploying Java Spring Boot applications, deploying to cloud platforms, and scaling applications to handle high traffic.
Hire Dedicated Developers for various technologies such as Android, Angular, React, IOS, PHP, Node, Joget, MongoDB, and Java with AppsRhino:
Contact AppsRhino Today!
Frequently Asked Questions (FAQs)
What is Java Spring Boot and how does it simplify web application development?
Java Spring Boot is a framework that simplifies the development of web applications by providing a convention-over-configuration approach and a range of ready-to-use modules, making it easier for developers to get started and build robust applications.
What are the advantages of using Java Spring Boot for web application development?
Some advantages of using Java Spring Boot include increased developer productivity due to its opinionated approach, automatic configuration, built-in security features, and seamless integration with other Spring projects.
How can I integrate a relational database into a Java Spring Boot application?
Java Spring Boot provides support for various relational databases through Spring Data JPA. By configuring the necessary dependencies and SQL scripts, you can easily connect, query, and persist data to a database of your choice.
Can I use Java Spring Boot to build RESTful APIs?
Yes, Java Spring Boot is well-suited for building RESTful APIs. It provides features like Spring MVC, which makes it easy to create REST endpoints, handle HTTP requests and responses, and serialize/deserialize data in various formats like JSON or XML.
Can I deploy a Java Spring Boot application to the cloud?
Yes, Java Spring Boot applications can be deployed to popular cloud platforms like AWS, Azure, or Heroku. These platforms provide deployment options, management tools, and scalability features that simplify the process of hosting and scaling Spring Boot applications.
How can I test and debug a Java Spring Boot application?
Java Spring Boot provides a range of tools for testing and debugging. You can use frameworks like JUnit or Mockito for unit testing, perform integration testing with Spring Test, and leverage built-in logging and debugging capabilities for troubleshooting and error identification.
Table of Contents
- Introduction
- What is Java Spring Boot?
- Benefits of using Java Spring Boot for web application development
- Setting up a Java Spring Boot project
- Building RESTful APIs with Java Spring Boot
- Working with Databases in Java Spring Boot
- Securing Java Spring Boot Applications
- Securing RESTful APIs with JSON Web Tokens (JWT)
- Integrating front-end frameworks with Java Spring Boot
- Conclusion
- Frequently Asked Questions (FAQs)