- Introduction
- What is PHP?
- Advantages of Using PHP
- Why Use PHP for Web Development?
- How to Set Up a PHP Development Environment
- Tips for Writing Efficient PHP Code
- Tricks for Optimizing PHP Performance
- Conclusion
- Frequently Asked Questions (FAQs)
Table of Contents
Getting Started with PHP Development: Tips and Tricks
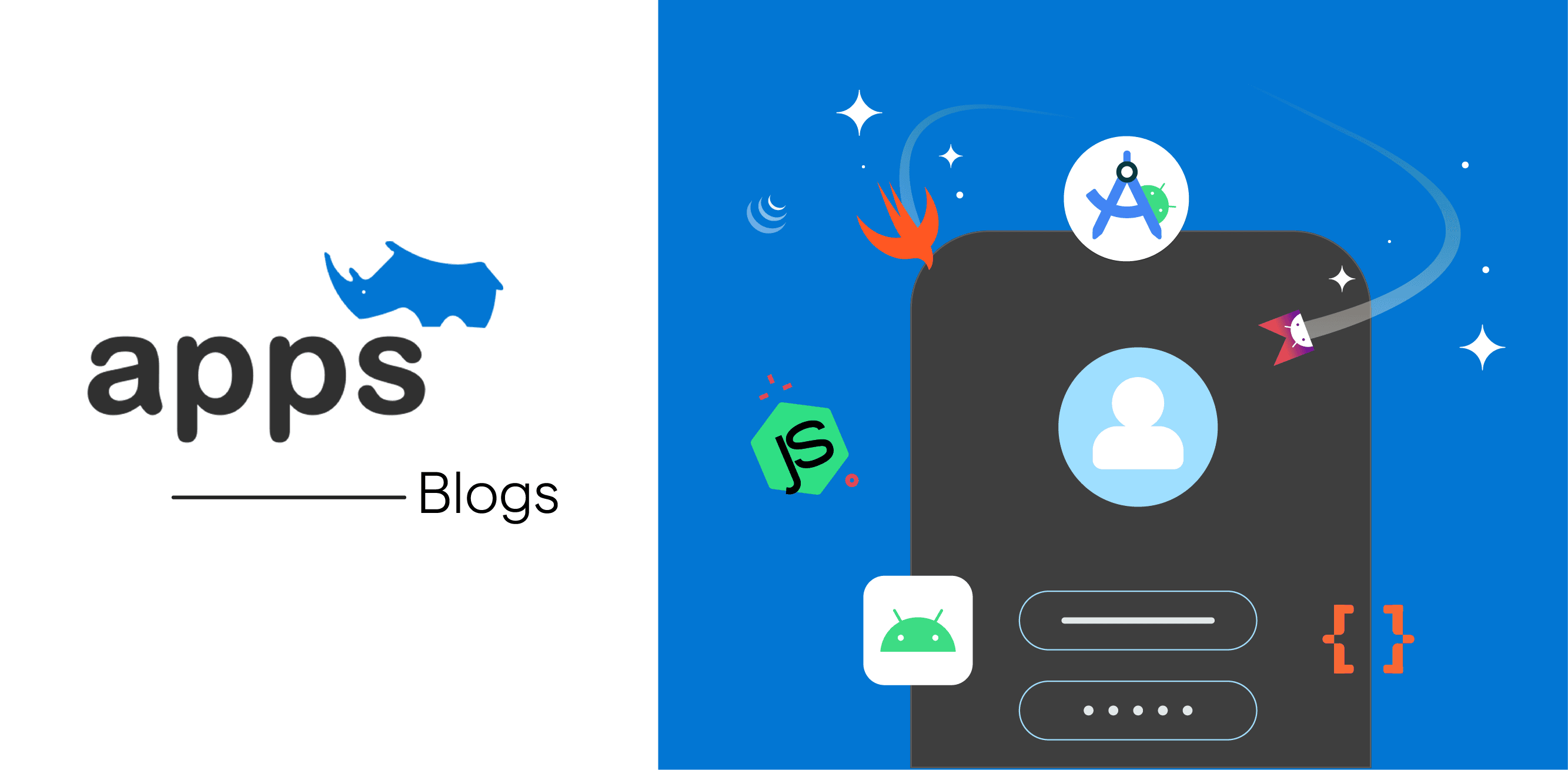
Introduction
Crossing the threshold of PHP development a person can either be a beginner looking to learn PHP or an experienced developer seeking to improve his PHP skills.
PHP is one of the most popular programming languages for web development, powering over 79% of all websites on the internet, supplying testament to its magnanimous audience.
Post coming across it ,questions might arise as to why is PHP so popular? Firstly a huge factor is played by the fact that it's free and open-source, which means anyone can use it without having to pay a dime.
It's also easy to learn, with a syntax that's similar to C and Java. And with a vast community of developers constantly contributing to its development, PHP is constantly evolving and improving.
Briefly shared below are some tips and tricks to help you get started with PHP development.
What is PHP?

PHP, which stands for Hypertext Preprocessor, is a server-side scripting language used for web development.
It is a popular choice among developers due to its versatility and ease of use. It is a server-side scripting language that is used to create dynamic web pages.
Being an open-source language, it means that it is free to use and can be modified by anyone.
PHP is designed to work with web servers, and it is used to create web applications, content management systems, and e-commerce websites.
Advantages of Using PHP

There are several advantages to using PHP for web development. Here are just a few:
Easy to Learn
PHP has a syntax that is similar to C and Java, making it easy for developers to learn.
It is also a forgiving language, which means that it is forgiving of errors and can still run even if there are mistakes in the code.
Open-Source
PHP is free and open-source, which means that anyone can use it without having to pay a dime.
This makes it an ideal choice for small businesses and startups that may not have a lot of money to invest in web development.
Versatile
PHP can be used for a wide range of web development tasks, from creating simple websites to building complex web applications.
It is also compatible with a variety of databases, including MySQL, PostgreSQL, and Oracle.
Large Community
PHP has a large community of developers constantly contributing to its development, which means it is constantly evolving and improving.
This community also provides support and resources for developers who are just starting out with PHP.
Why Use PHP for Web Development?

PHP is a popular server-side scripting language that is used by millions of developers around the world.
It is a great choice for web development due to its ease of use, versatility, and compatibility with a variety of databases.
PHP is also free and open-source, making it an ideal choice for small businesses and startups that may not have a lot of money to invest in web development.
Additionally, PHP has a large community of developers constantly contributing to its development, which means it is constantly evolving and improving.
Overall, PHP is a powerful and versatile language that is definitely worth considering for web development projects.
Suggested Reading:
How to Set Up a PHP Development Environment

Whether interested in learning PHP or developing web applications using PHP, they’ll need to set up a PHP development environment.
In this blog post, the steps to set up a PHP development environment on your computer are:
Step 1
Install a Web Server
The first step in setting up a PHP development environment is to install a web server.
A web server is a software application that runs on your computer and serves web pages to users who request them. There are several web servers available, but the most popular one is Apache.
To install Apache, you can download it from the Apache website and follow the installation instructions.
Once you have installed Apache, you can test it by opening a web browser and navigating to http://localhost/. If Apache is installed correctly, you should see a message that says "It works!".
Step 2
Install PHP
The next step is to install PHP. It is a server-side scripting language that is used to create dynamic web pages.
To install PHP, you can download it from the PHP website and follow the installation instructions.
Once you have installed PHP, you can test it by creating a PHP file and placing it in the Apache web server's document root directory.
The document root directory is the directory on your computer where Apache looks for web pages to serve.
To create a PHP file, open a text editor and type the following code:
php
Copy
<?php
echo "Hello, World!";
?>
Save the file as "index.php" and place it in the document root directory. Then, open a web browser and navigate to http://localhost/index.php. If PHP is installed correctly, you should see the message "Hello, World!".
Step 3:
Install a Database
If you're planning to develop web applications that require a database, you'll need to install a database management system. The most popular database management system for PHP is MySQL.
To install MySQL, you can download it from the MySQL website and follow the installation instructions. Once you have installed MySQL, you can test it by creating a database and a table.
To create a database, open a command prompt and type the following command:
Bash Copy mysql -u root - p
|
This will open the MySQL command-line interface. Then, type the following commands to create a database and a table:
mysql
Copy
CREATE DATABASE mydatabase;
USE mydatabase;
CREATE TABLE mytable (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
firstname VARCHAR(30) NOT NULL,
lastname VARCHAR(30) NOT NULL,
email VARCHAR(50),
reg_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
Tips for Writing Efficient PHP Code

PHP is a powerful and versatile server-side scripting language that is used by millions of developers around the world.
However, writing efficient PHP code can be a challenge, especially for beginners. Some tips for writing efficient PHP code are:
Use Proper Coding Standards
Using proper coding standards is essential for writing efficient PHP code. It makes a code more readable and easier to maintain.
Some popular coding standards for PHP include PSR-1, PSR-2, and PSR-12. These standards cover topics such as naming conventions, indentation, and code structure.
Suggested Reading:
Why should you choose AppsRhino For PHP Based Projects?
Avoid Using Global Variables
Global variables can make your code harder to read and maintain. They can also cause unexpected behavior if they are modified in one part of your code and used in another.
Instead, use local variables or pass variables as function parameters.
Optimize Database Queries
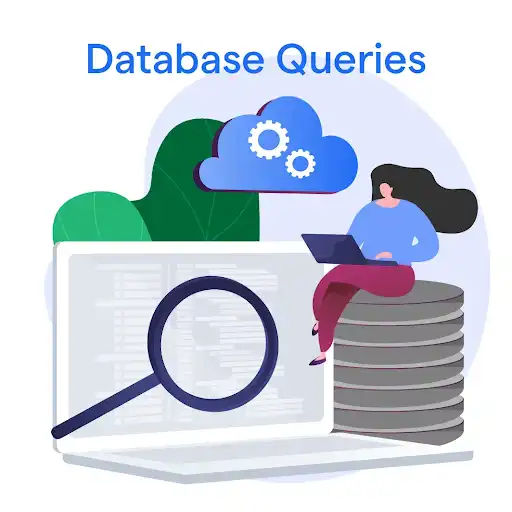
Database queries can be a significant bottleneck in PHP applications.
To optimize database queries, you can use indexes, limit the number of rows returned, and use prepared statements to prevent SQL injection attacks.
Use Caching
Caching can significantly improve the performance of your PHP code.
Caching involves storing the results of expensive operations, such as database queries, in memory or on disk.
This allows you to retrieve the results quickly without having to perform the operation again.
Use Debugging Tools

Debugging tools can help you identify and fix errors in your PHP code. Some popular debugging tools for PHP include Xdebug, Zend Debugger, and PHP Debug Bar.
These tools allow you to step through your code, set breakpoints, and view variables and function calls.
Use Functions and Classes
Using functions and classes can make the code more modular and easier to maintain.
Functions allow you to break your code into smaller, reusable pieces, while classes allow you to encapsulate data and behavior into objects.
Suggested Reading:
Node.js vs. PHP: Which Is Better for Your App Development Needs?
Use Built-in Functions
PHP has a large number of built-in functions that can save you time and effort when writing code.
These functions include string manipulation functions, array functions, and date and time functions.
Using built-in functions can also make your code more efficient, as they are often optimized for performance.
Tricks for Optimizing PHP Performance

In order to upgrade your overall performance certain tips and tricks can be put to use which are listed below:
Use Opcode Caching
Opcode caching is a technique that involves storing compiled PHP code in memory, so it can be executed more quickly.
This can significantly improve the performance of PHP applications, especially if they use a lot of PHP code. Some popular opcode caching tools for PHP include APC and OpCache.
Use a Content Delivery Network (CDN)

A content delivery network (CDN) is a network of servers that are distributed around the world.
By using a CDN, you can serve your PHP applications from a server that is geographically closer to your users, which can significantly reduce the time it takes for your applications to load.
Some popular CDNs for PHP applications include Cloudflare and Akamai.
Use Gzip Compression
Gzip compression is a technique that involves compressing the output of your PHP applications before sending it to the user's browser.
This can significantly reduce the amount of data that needs to be transferred, which can improve the performance of your applications. To enable Gzip compression in PHP development, you can use the zlib extension.
Use a PHP Accelerator
A PHP accelerator is a tool that can improve the performance of your PHP applications by caching the compiled PHP code in memory.
Some popular PHP accelerators include APC and OpCache. These tools can significantly improve the performance of your PHP applications, especially if they use a lot of PHP code
Optimize Database Queries
Database queries can be a significant bottleneck in PHP applications.
To optimize database queries, you can use indexes, limit the number of rows returned, and use prepared statements to prevent SQL injection attacks.
You can also use a tool like MySQLTuner to analyze your MySQL database and suggest optimizations.
Conclusion
In conclusion, PHP is a powerful and versatile server-side scripting language that is used by millions of developers around the world.
It is a great choice for web development due to its ease of use, versatility, and compatibility with a variety of databases.
Additionally, being free and open-source, and having a large community of developers constantly contributing to its development, make it an attractive option for small businesses and startups.
To get the most out of your PHP applications, it's important to follow proper coding standards, avoid global variables, use functions and classes, use built-in functions, use caching, optimize database queries, and use debugging tools.
Additionally, optimizing PHP performance requires using opcode caching, using a content delivery network (CDN), using Gzip compression, using a PHP accelerator, optimizing database queries, and using a content management system (CMS).
By following these tips and tricks, one can write efficient PHP code and optimize the performance of PHP applications, making them faster and more responsive for users. Whether being a beginner or an experienced developer.
Suggested Reading:
Hiring PHP developers from AppsRhino is wise: Here's why!
Frequently Asked Questions (FAQs)
What is PHP?
PHP stands for Hypertext Preprocessor. It is a popular open-source scripting language used for web development. It is widely used to create dynamic web pages and web applications.
How does PHP differ from other programming languages?
PHP is a server-side programming language, which means it runs on a web server and generates HTML output that can be viewed in a browser.
Other programming languages, such as JavaScript, run on a client's web browser. Additionally, PHP is a beginner-friendly language that is easier to learn compared to other programming languages such as Java or Python.
What are some common use cases of PHP?
PHP is commonly used for web development tasks, specifically server-side scripting, web services, and creating robust web applications.
It is most widely used to develop e-commerce sites, content management systems (CMS), social networking sites, and web-based business applications.
Do I need to install PHP to develop PHP applications?
Yes, you need to have PHP installed on your computer to develop PHP applications. You can install PHP on most web servers, or you can download it on your local computer to create web applications locally.
What tools can be used for PHP development?
PHP can be developed on any plain-text editor. However, many PHP developers use integrated development environments (IDEs) for improved development efficiency.
Some popular PHP IDEs are PhpStorm, Sublime Text, and Visual Studio Code.
How can I learn PHP development?
There are a wide variety of online resources available to learn PHP development, including official PHP documentation, free and paid online courses, instructional videos on YouTube, and online learning platforms such as Udemy, Coursera, and edX.
Many developers also recommend building real-world projects to gain practical experience and confidence in PHP development.
Table of Contents
- Introduction
- What is PHP?
- Advantages of Using PHP
- Why Use PHP for Web Development?
- How to Set Up a PHP Development Environment
- Tips for Writing Efficient PHP Code
- Tricks for Optimizing PHP Performance
- Conclusion
- Frequently Asked Questions (FAQs)